Build For Yourself: Automating Workflows as a Developer
I love to automate my workflows. I've been doing it for years, and it's improving not only my productivity, but also the quality and satisfaction of my work.
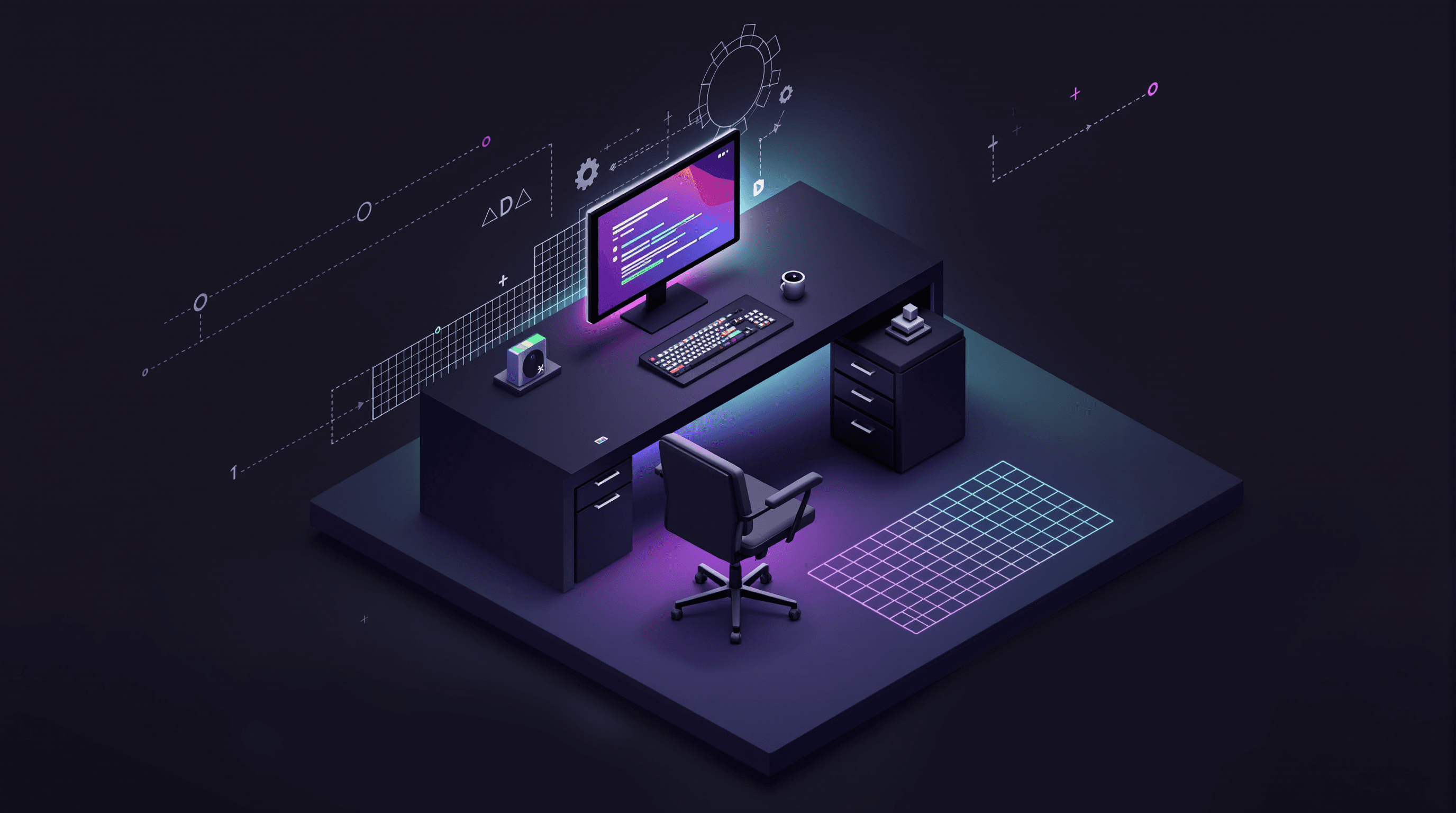
Why Automate?
Imagine this: you're in a middle of your focused work, trying out different ideas, and then you hit an error: botocore.exceptions.ClientError: An error occurred (ExpiredToken)
. You're thinking "Oh right, I forgot to refresh my AWS credentials". You open a terminal, run aws sso login
, go to browser to allow the access, probably you'll also have to restart your server. It took you less than a minute to do all this, but now you're asking yourself "So what was the idea I had in mind?".
As engineers, we create most of the value during the uninterrupted, deep work (often in flow state). But our processes are inevitably surrounded by a lot of repetitive, shallow tasks. It slows us down, but what's worse is that it can break our flow and focus. This can lead to wasted time, forgotten ideas, and decreased enjoyment of the work.
At the same time, as engineers, we are in a unique position to automate a lot of shallow tasks. We can create scripts, extensions, and much more, just for ourselves.
Idea
When I started writing this article, I had a huge list of productivity hacks and tips, but I realized that most of them are not about automation, but more about improving your workflow. I guess I'll make another article about that, but for now, let's focus on automation.
The common idea behind most productivity improvements is that you should accelerate your workflow as close to the speed of thought as possible.
We'll start from simple, general tools and then move to more specific ad-hoc ones.
Hotkeys
While not automation per se, hotkeys are great entrypoints for your automations.
In my hyperkey article, I've already covered how you can speed up small tasks, like opening apps or running common commands.
This is pretty minor, but remember that it's not just about the time you save, but also about the mental space you free up.
Shell: Aliases and Functions
Why use git
when you can use g
? For more advanced use cases with arguments, you can use functions.
Nowadays, 99% of all the commands in my shell are custom aliases/functions.
Tip #1: check your shell history (~/.bash_history
or ~/.zsh_history
) to see what you use the most and then create an alias for it.
Tip #2: use AI to create these little helpers. You can even send some parts of your shell history and ask it to come up with some optimizations for you.
Here's an example of a few git
-related functions I use:
function g-rm-stale {
git fetch -p
for branch in $(git branch -vv | grep ': gone]' | awk '{print $1}'); do git branch -D $branch; done
}
function gmp {
local branch
if git show-ref --verify --quiet refs/heads/main; then
branch="main"
elif git show-ref --verify --quiet refs/heads/production; then
branch="production"
else
branch="master"
fi
current_branch=$(git rev-parse --abbrev-ref HEAD)
if [ "$current_branch" = "$branch" ]; then
git pull origin $branch
else
git fetch origin $branch:$branch
git checkout $branch
fi
g-rm-stale
}
Develop for Development
One of the underrated ways to improve your productivity is to build tools around the development process itself.
We are familiar with the development environment, for example, when running a server in development mode with hot reloading. But you can do much more than that.
To stay in the flow, you need to have a short feedback loop, but with complex systems, it can take a lot of time to get a result, so you may want to build workarounds to quickly check your changes.
"Oh look, he invented unit tests!", you might say. Well, you may not want to have a unit test for certain things, e.g. because of fuzzy outputs or complexity of the involved systems.
But do create tests. Please.
Snippets
This is somewhat similar to aliases, but more oriented towards pasting text. Do you often have to input the same text in different places? Create a snippet!
Raycast, one of my favorite productivity tools, has a great snippets feature. You can create snippets with dynamic variables, assign aliases to it. I use it for things like emails, names and other frequently used text.
For more dev-oriented snippets, it's better to use your IDE, since it will have a better support for code pasting and multiple cursors.
For example, when debugging some scraping scripts, you might want to quickly add code to save HTTP response to a file. You may create a snippet that looks like this:
with open('response.json', 'wb') as f:
f.write(response.content)
Postman Automation
This one is a bit niche, but if you're often interacting with APIs, you can find a lot of value in Postman and its automation features.
For example, I had to often make requests to the API with dynamic token located in a JSON file in my filesystem. Postman doesn't allow you to access the filesystem directly, but you can spin up a local server and use it to get the token.
# start a local server
python -m http.server 8136
And then in Postman, you can set up a pre-request script (you can do this both in the collection and in the request level) to get the token from the server.
pm.sendRequest("http://localhost:8136/request.json", function (err, response) {
const data = response.json();
pm.collectionVariables.set('bearertoken', data.auth_token)
});
And then just use {{bearertoken}}
whenever you need it, for example in the Authorization tab of your collection:
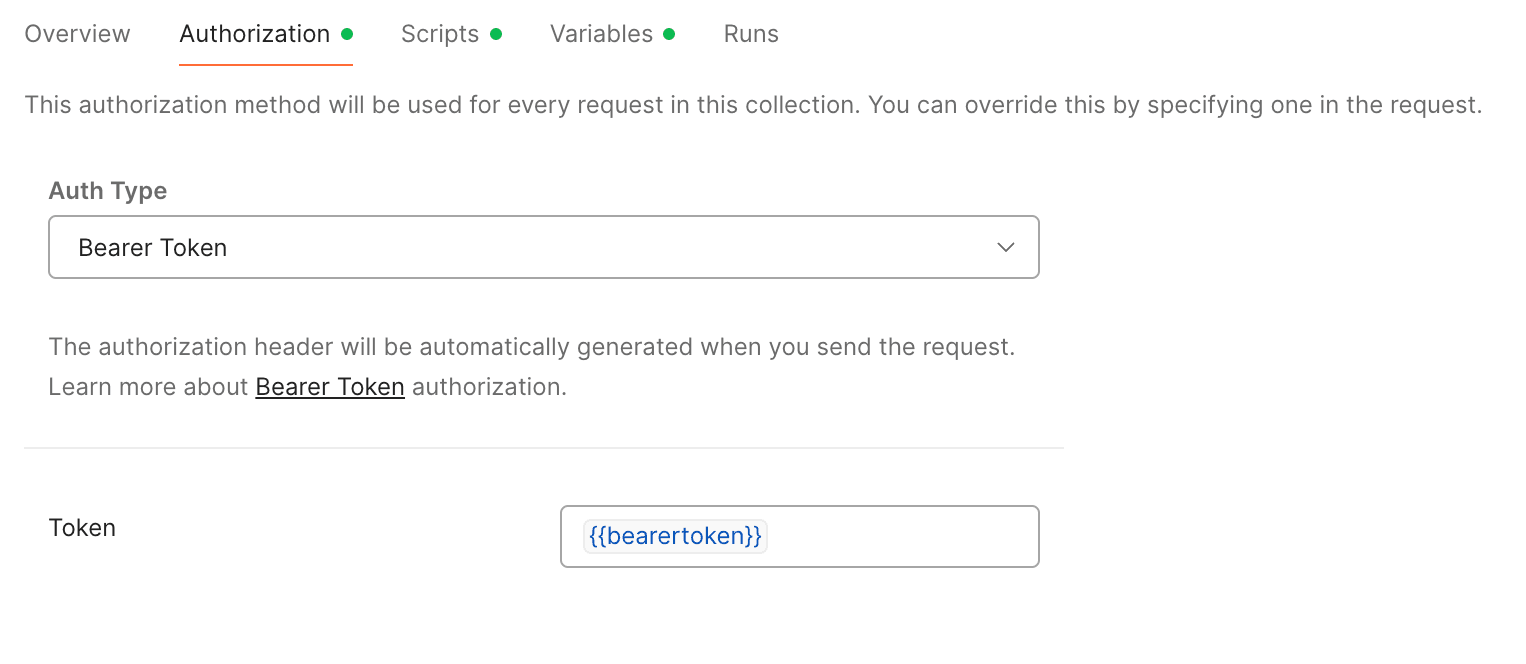
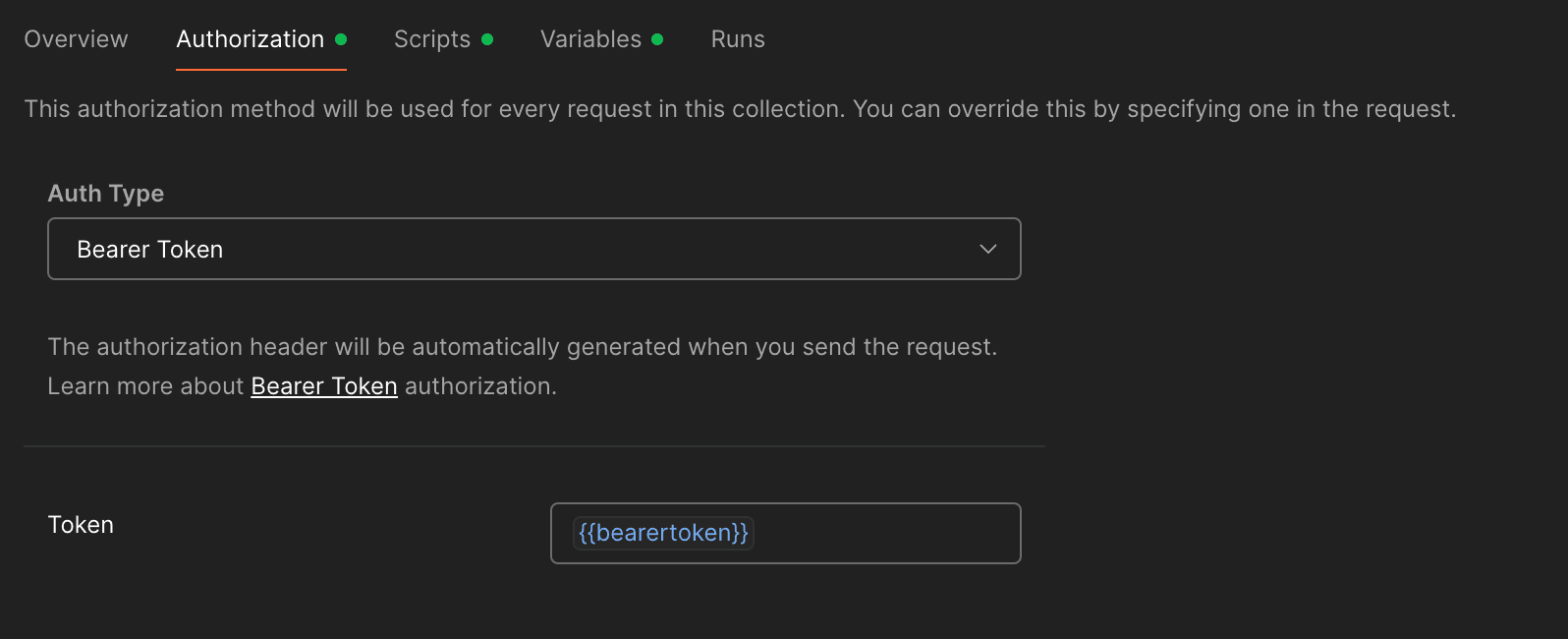
Tip: if you need to auto-copy values from responses to your clipboard, you can hack it with a custom visualizer.
Other Tools
Browser and IDE Extensions
Custom browser extensions and IDE plugins can be useful, but they usually require more effort and knowledge to create, and if not done correctly, it can actually slow you down.
AI Prompting
I must mention this, because GPT models are getting integrated into developers' workflows more and more. It can help you with writing not only code and documentation, but also PR descriptions, commit messages, copy (e.g. the text in popups/dialogs). My main recommendation is still to use Cursor IDE.
Conclusion
I hope this article will give you some ideas on how you can improve your productivity by automating your workflows.
If you have any questions or suggestions, feel free to reach out to me.